1、程序簡介
該程序是基于OpenHarmony標準系統的C++公共基礎類庫的線程池處理:ThreadPoll。
本案例完成如下工作:
創建1個線程池,設置該線程池內部有1024個線程空間。
啟動5個線程。每個線程每秒打印1段字符串,10秒后停止。
2、基礎知識
C++公共基礎類庫為標準系統提供了一些常用的C++開發工具類,包括:
文件、路徑、字符串相關操作的能力增強接口
安全數據容器、數據序列化等接口
各子系統的錯誤碼相關定義
2.1、添加C++公共基礎類庫依賴
修改需調用模塊的BUILD.gn,在external_deps或deps中添加如下:
ohos_shared_library("xxxxx") { ... external_deps = [ ... # 動態庫依賴(可選) "c_utils:utils", # 靜態庫依賴(可選) "c_utils:utilsbase", # Rust動態庫依賴(可選) "c_utils:utils_rust", ] ...}
一般而言,我們只需要填寫"c_utils:utils"即可。
2.2、ThreadPoll頭文件
ThreadPoll提供線程安全的線程池功能。
ThreadPoll維護一個任務隊列,一個線程組。開發者只需向任務隊列中注冊需要進行的任務,線程組執行任務隊列中的任務。
C++公共基礎類庫的Thread頭文件在://commonlibrary/c_utils/base/include/thread_pool.h
可在源代碼中添加如下:
#include
命令空間如下:
OHOS::ThreadPool
2.3、OHOS::Thread接口說明
thread_ex.h定義Thread類,該類負責定義Thread類以及相關接口。
2.3.1、ThreadPool
構造函數, 構造ThreadPool對象,為線程池內線程命名。
explicit ThreadPool(const std::string &name = std::string());
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
name | std::string | 線程名稱 |
2.3.2、~ThreadPool
析構函數。
~ThreadPool();
2.3.3、AddTask
向任務隊列中添加一個Task。若未調用Start()則直接執行Task且不會向任務隊列添加該Task。
void AddTask(const Task& f);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
f | std::function | 函數 |
2.3.4、GetCurTaskNum
獲取當前任務數。
size_t GetCurTaskNum();
返回值說明:
類型 | 返回值說明 |
---|---|
size_t | 返回當前任務數 |
2.3.5、GetMaxTaskNum
獲取最大任務數。
size_t GetMaxTaskNum() const;
返回值說明:
類型 | 返回值說明 |
---|---|
size_t | 獲取最大任務數 |
2.3.6、GetName
獲取線程池命名。
std::string GetName() const;
返回值說明:
類型 | 返回值說明 |
---|---|
std::string | 線程池命名名稱 |
2.3.7、GetThreadsNum
獲取線程池內線程數。
size_t GetThreadsNum() const;
返回值說明:
類型 | 返回值說明 |
---|---|
size_t | 獲取線程池內線程數 |
2.3.8、SetMaxTaskNum
設置任務隊列中最大任務數。
void SetMaxTaskNum(int maxSize);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
maxSize | int | 最大任務數 |
2.3.9、Start
啟動給定數量threadsNum的線程,執行任務隊列中的任務。
uint32_t Start(int threadsNum);
參數說明:
參數名稱 | 類型 | 參數說明 |
---|---|---|
threadsNum | int | 需要啟動線程的數量 |
返回值說明:
返回值數值 | 返回值說明 |
---|---|
ERR_OK | 成功 |
其它 | 錯誤 |
2.3.10、Stop
停止線程池,等待所有線程結束。
void Stop();
3、程序解析
3.1、創建編譯引導
在samples/BUILD.gn文件添加一行編譯引導語句。
import("http://build/ohos.gni")
group("samples") { deps = [ "a24_utils_thread_poll:utils_threadpoll", # 添加該行 ]}
"samples/a24_utils_thread_poll:utils_threadpoll",該行語句表示目錄源代碼 參與編譯。
3.2、創建編譯項目
創建samples/a24_utils_thread_poll 目錄,并添加如下文件:
a24_utils_thread_poll├── utils_thread_poll_sample.cpp # .cpp源代碼├──BUILD.gn#GN文件
3.3、創建BUILD.gn
編輯BUILD.gn文件。
import("http://build/ohos.gni")ohos_executable("utils_threadpoll") { sources = [ "utils_thread_poll_sample.cpp" ] include_dirs = [ "http://commonlibrary/c_utils/base/include", "http://commonlibrary/c_utils/base:utils", "http://third_party/googletest:gtest_main", "http://third_party/googletest/googletest/include" ] external_deps = [ "c_utils:utils" ] part_name = "product_rk3568" install_enable = true}
注意:
(1)BUILD.gn中所有的TAB鍵必須轉化為空格,否則會報錯。如果自己不知道如何規范化,可以:
# 安裝gn工具sudo apt-get install ninja-buildsudo apt install generate-ninja# 規范化BUILD.gngn format BUILD.gn
3.4、創建源代碼
3.4.1、創建線程池
引用頭文件,定義OHOS::ThreadPool類對象(即創建線程池)。
#include // 線程池的頭文件
int main(int argc, char **argv){ OHOS::ThreadPool thread_poll("thread_poll_name"); ......}
3.4.2、獲取和設置線程池最大任務數
通過ThreadPool.GetMaxTaskNum()函數獲取線程池最大任務數。
通過ThreadPool.SetMaxTaskNum()函數設置線程池最大任務數。
具體代碼如下:
int main(int argc, char **argv){ ...... // 查看默認的線程池最大任務數 cout << "get max task num(default): " << thread_poll.GetMaxTaskNum() << endl; // 設置線程池的最大任務數 cout << "set max task num: " << max_task_num << endl; thread_poll.SetMaxTaskNum(max_task_num); // 再查看線程池最大任務數 cout << "get max task num(set): " << thread_poll.GetMaxTaskNum() << endl; ......}
3.4.3、啟動線程池并添加線程
通過ThreadPool.Start()函數啟動線程池線程。
通過ThreadPool.AddTask()函數添加線程,并設置執行函數。
具體代碼如下:
int main(int argc, char **argv){ ...... // 開啟啟動線程 cout << "start thread: " << start_task_num << endl; thread_poll.Start(start_task_num); for (i = 0; i < start_task_num; i++) { cout << "add task: i = " << i << endl; str_name = "thread_pool_" + to_string(i); auto task = std::bind(func, str_name); // 添加任務到線程池中,并啟動運行 thread_poll.AddTask(task); sleep(1); } ......}
3.4.4、編寫線程執行函數
每秒打印一段信息,10秒后退出。
void func(const std::string &name){ for (int i = 0; i < 10; i++) { cout << "func: " << name << " and i = " << i << endl; sleep(1); }}
3.4.5、主程序等待線程池全部退出
通過ThreadPool.Start()函數啟動線程池線程。
具體代碼如下:
int main(int argc, char **argv){ // 等待關閉所有的線程,會等待線程池程序全部結束才返回 cout << "stop thread: start" << endl; thread_poll.Stop(); cout << "stop thread: end" << endl; return 0;}
4、運行程序
系統啟動后,運行命令:
utilsthreadpoll
5、運行結果
運行結果:
# utils_threadpollget max task num(default): 0set max task num: 1024get max task num(set): 1024start thread: 5add task: i = 0func: thread_pool_0 and i = 0add task: i = 1func: thread_pool_0 and i = 1func: thread_pool_1 and i = 0add task: i = 2func: thread_pool_0 and i = 2func: thread_pool_1 and i = 1func: thread_pool_2 and i = 0add task: i = 3func: thread_pool_0 and i = 3func: thread_pool_1 and i = 2func: thread_pool_3 and i = 0func: thread_pool_2 and i = 1func: thread_pool_0 and i = 4func: thread_pool_3 and i = 2add task: i = 1func: thread_pool_2 and i = 42func: thread_pool_1 and i = 3func: thread_pool_4 and i = 0func: thread_pool_0 and i = 5func: thread_pool_3 and i = 2func: thread_pool_1 and i = 4func: thread_pool_2 and i = 3stop thread: startfunc: thread_pool_4 and i = 1func: thread_pool_0 and i = 6func: thread_pool_1 and i = 5func: thread_pool_3 and i = 3func: thread_pool_4 and i = 2func: thread_pool_2 and i = 4func: thread_pool_0 and i = 7func: thread_pool_1 and i = 6func: thread_pool_3 and i = 4func: thread_pool_2 and i = 5func:thread_pool_4 and i = 3func: thread_pool_0 and i = 8func: thread_pool_1 and i = 7func: thread_pool_3 and i = 5func: thread_pool_2 and i = 6func: thread_pool_4 and i = 4func: thread_pool_0 and i = 9func: thread_pool_1 and i = 8func: thread_pool_3 and i = 6func: thread_pool_2 and i = func: thread_pool_4 and i = 57func: thread_pool_1 and i = 9func: thread_pool_3 and i = 7func: thread_pool_4 and i = 6func: thread_pool_2 and i = 8func: thread_pool_3 and i = 8func: thread_pool_4 and i = 7func: thread_pool_2 and i = 9func: thread_pool_3 and i = 9func: thread_pool_4 and i = 8func: thread_pool_4 and i = 9stop thread: end#
注意:
(1)因有10個線程做出打印信息,故上述打印信息各有不同。
-
線程
+關注
關注
0文章
507瀏覽量
20067 -
OpenHarmony
+關注
關注
26文章
3817瀏覽量
18090
發布評論請先 登錄
基于OpenHarmony標準系統的C++公共基礎類庫案例:ThreadPoll
基于OpenHarmony標準系統的C++公共基礎類庫案例:Semaphore
基于OpenHarmony標準系統的C++公共基礎類庫案例:rwlock
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeQueue
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeStack
OpenHarmony C++公共基礎類庫應用案例:Thread
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeBlockQueue
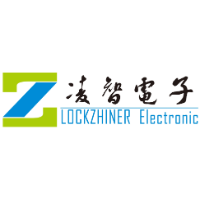
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeStack
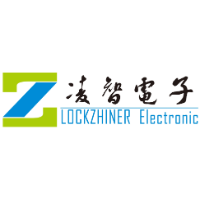
基于OpenHarmony標準系統的C++公共基礎類庫案例:SafeQueue
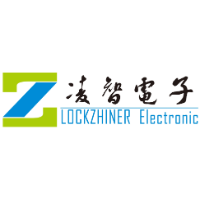
基于OpenHarmony標準系統的C++公共基礎類庫案例:rwlock
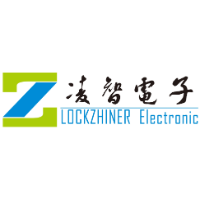
評論